A/B Testing and Feature Management
Our SDK helps you to decouple product experiences from code on your app in real-time with product variables. These product variables lets you change the behavior as well as the appearance of the app without requiring users to download an app update.
When using this feature, you essentially create in-app product variables and their default values that control the behavior and appearance of the app. You can later use the Hansel dashboard to change these default values and update product variables for all or segments of your app user base.
This guide will help you with the steps related to product variables. Product variables for a given feature are configs having a key name, default value, and defined data type.
Type of product variables
Let's first understand product variables and their types.
Type of product variable | What is it? | When to choose this? |
---|---|---|
String | This product variable is simply a collection of characters and numbers which helps you to perform text-based changes in run-time. | Whenever you are customizing the content of your app or website, you can choose string product variable type. |
Number | Number product variables are used to define decimal or integer values. | If you are about to make numeric changes in your app or website, select a Number product variable type. |
Boolean | This product variable is used to represent one of the two values - True or False. | When you to want to turn OFF/ ON the features and functions of your app or website, do it in one stroke with a Boolean product variable type. |
JSON | This product variable is a collection of Key-Value pairs used to define an object for structuring data. | You can use JSON product variable type for managing entire data sets from Hansel dashboard in order to store and organize site or app content for e.g while creating a homepage. |
Step 1. Create product variables on dashboard
1: Head over to the Product Variables section from the left menu
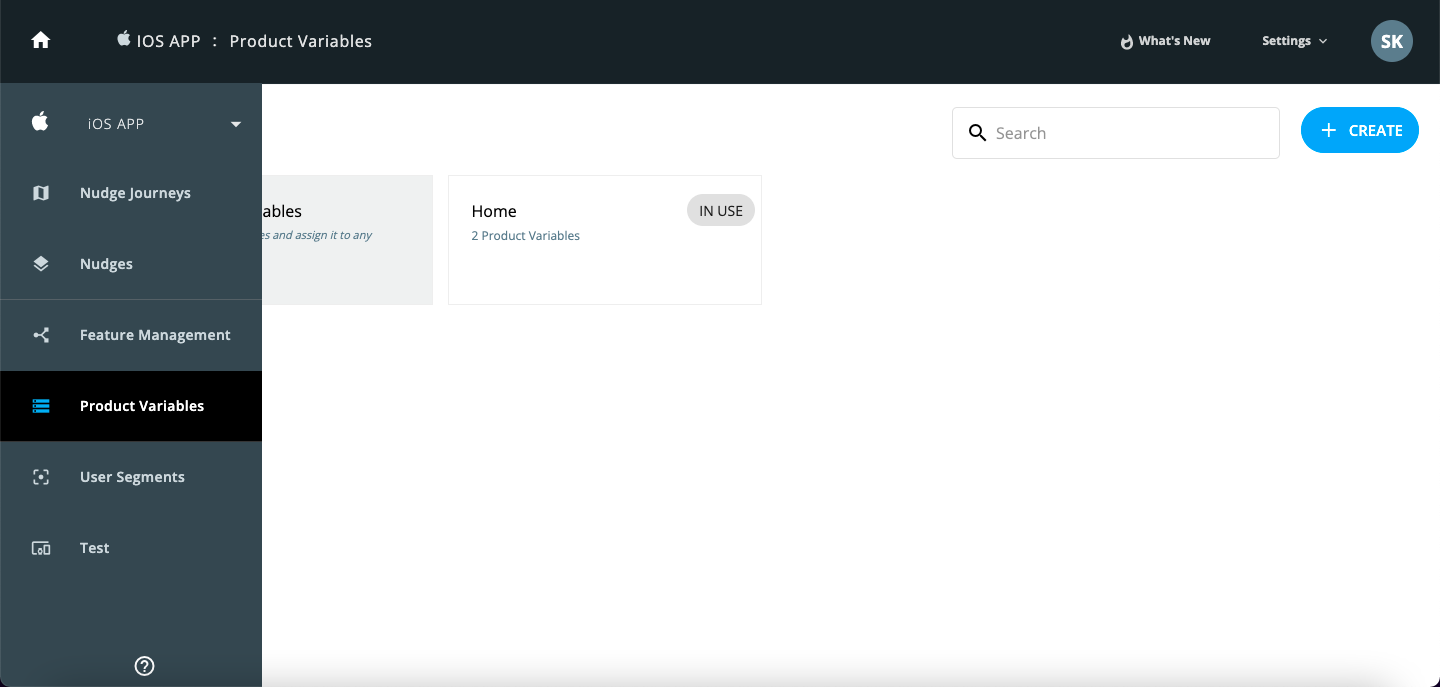
2: Click on Create Feature Module button. A feature module refers to a particular feature where you want to define certain product variables. As an example, let's take the example of "Wishlist" as a feature module.
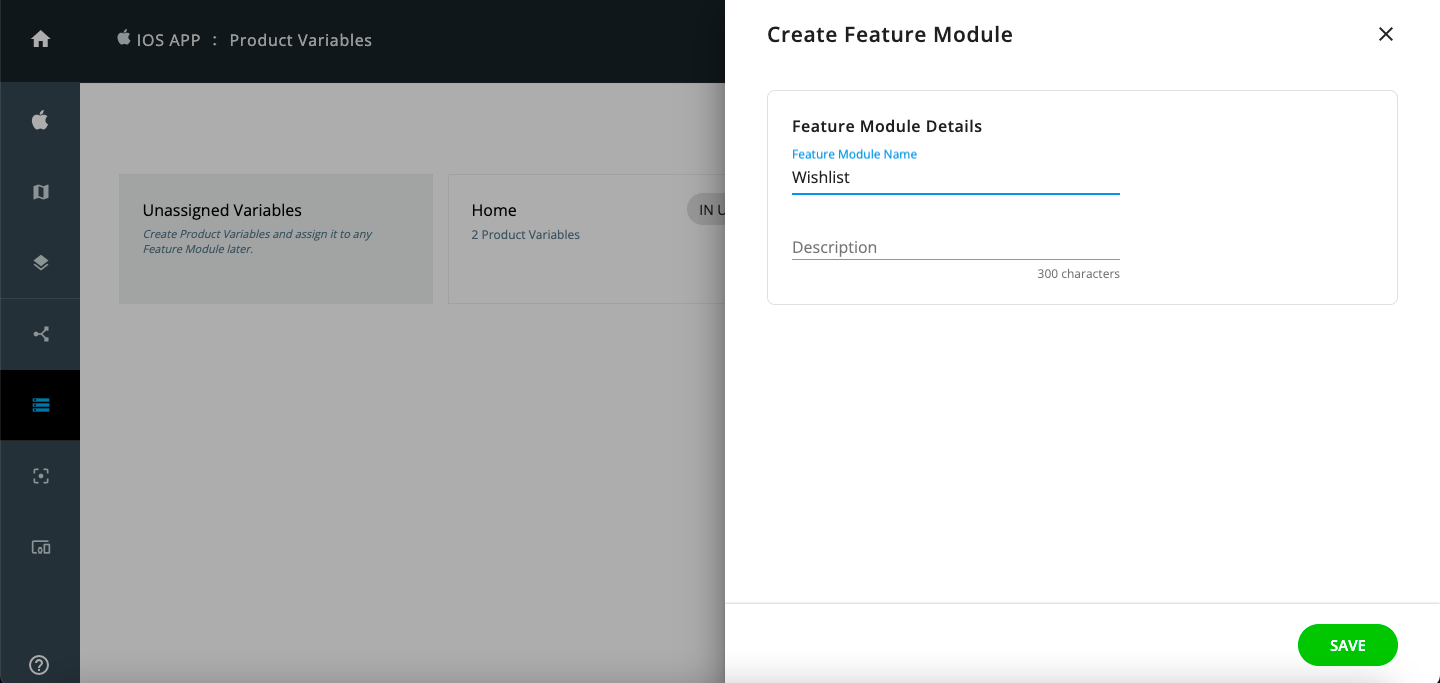
3: Once you are done creating a feature module, you can start adding product variables. Product variable consists of a key name, the data type of its value such as string, number, boolean, and json, and the default value for this key.
Continuing our example of "Wishlist" feature module, the below screenshot shows how we can add product variable "wishlist_feature_enabled" having boolean datatype and default value as false.
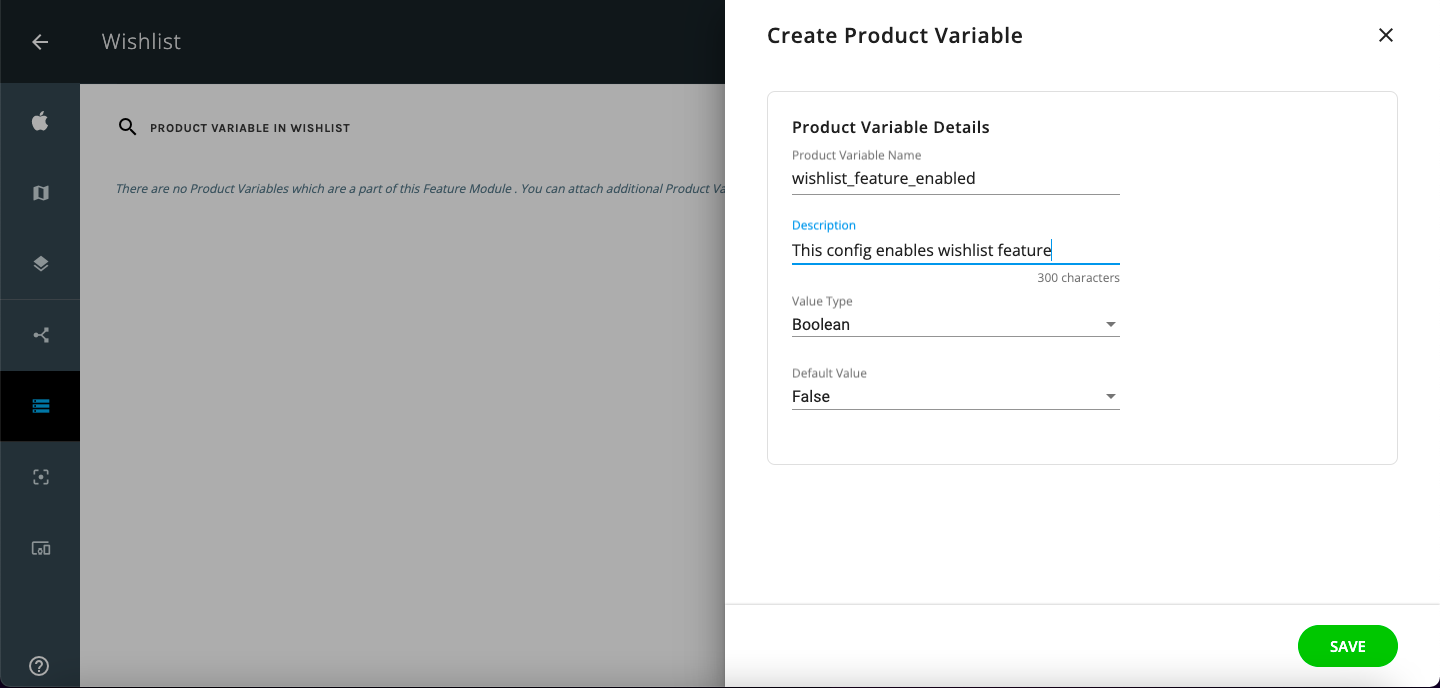
- In a similar manner, you can add multiple product variables to the given feature module.
- Otherwise, you can also create unassigned product variables, and add them to any already added feature module later on.
- You can also optionally enter a short “Description” for these product variables.
Step 2. Using product variables from the dashboard in your app
Once you are done with the above step of creating the product variables, you need to implement the below code snippets based on the applicable data type and relevant feature module where you want to add these product variables.
Note
Please take a note following parameters when it comes to adding below mentioned code snippets to your app
- key : This is the name of the product variable on the Hansel Dashboard
- fallbackValue(optional) : This is a fallback value to use if the key doesn't exist or the connection with the Netcore backend hasn't been established. Although fallback value is optional it is recommended that you provide one.
1. Fetching the boolean config
Parameters
- key : name of the deep config on the Hansel Dashboard
- fallbackValue(optional) : fall back value to use if the key doesn't exist or connection with the Hansel hasn't been established. Although fallback value is optional it is recommended that you provide one.
Return Value
boolean OR fallbackValue if provided OR nil/optional if no fallback value is provided.
#import <Hansel/Hansel-umbrella.h>
//Use the deep config you created on the dashboard
BOOL featureEnabled = [HanselConfigs getBool:@"config_name_here" withDefaultValue:false];
//Use featureEnabled to show/hide the feature
//Use the deep config you created on the dashboard
let featureEnabled = HanselConfigs.getBool("config_name_here", withDefaultValue:false)
//Use featureEnabled to show/hide the feature
2. Fetching the string config
Parameters
- key : name of the deep config on the Hansel Dashboard
- fallbackValue(optional) : fall back value to use if the key doesn't exist or connection with the Hansel hasn't been established. Although fallback value is optional it is recommended that you provide one.
Return Value
string OR fallbackValue if provided OR nil/optional if no fallback value is provided.
#import <Hansel/Hansel-umbrella.h>
//Use the deep config you created on the dashboard
NSString* featureName = [HanselConfigs getString:@"config_name_here" withDefaultValue:"Default String"];
//Use the deep config you created on the dashboard
let featureName = HanselConfigs.getString("config_name_here", withDefaultValue:"Default String")
3. Fetching the Double or Number config
Parameters
- key : name of the deep config on the Hansel Dashboard
- fallbackValue(optional) : fall back value to use if the key doesn't exist or connection with the Hansel hasn't been established. Although fallback value is optional it is recommended that you provide one.
Return Value
double/Double OR fallbackValue if provided OR nil/optional if no fallback value is provided.
#import <Hansel/Hansel-umbrella.h>
//Use the deep config you created on the dashboard
double featureValue = [HanselConfigs getDouble:@"config_name_here" withDefaultValue:10.0];
//Use the deep config you created on the dashboard
let featureValue = HanselConfigs.getDouble("config_name_here", withDefaultValue:10.0)
4. Fetching the JSON config
Parameters
- key : name of the deep config on the Hansel Dashboard
- fallbackValue(optional) : fall back value to use if the key doesn't exist or connection with the Hansel hasn't been established. Although fallback value is optional it is recommended that you provide one.
Return Value
JSON OR fallbackValue if provided OR nil/optional if no fallback value is provided.
#import <Hansel/Hansel-umbrella.h>
//Use the deep config you created on the dashboard
NSDictionary* json = [HanselConfigs getJSON:@"config_name_here" withDefaultValue:@{@"default key":@"default json"}];
//Use the deep config you created on the dashboard
let json = HanselConfigs.getJSON("json", withDefaultValue: ["default Key":"default Value"])
5. Fetching the JSON Array config
Parameters
- key : name of the deep config on the Hansel Dashboard
- fallbackValue(optional) : fall back value to use if the key doesn't exist or connection with the Hansel hasn't been established. Although fallback value is optional it is recommended that you provide one.
Return Value
JSON Array OR fallbackValue if provided OR nil/optional if no fallback value is provided.
#import <Hansel/Hansel-umbrella.h>
//Use the deep config you created on the dashboard
NSArray* jsonArray = [HanselConfigs getJSONArray:@"config_name_here" withDefaultValue:@[@"default listval1",@"default listval2"]];
//Use the deep config you created on the dashboard
let jsonArray = HanselConfigs.getJSONArray("config_name_here", withDefaultValue: [["defauly Key1":"default Value1"],["defauly Key2":"default Value2"]])
Step 3: Creating your first A/B test and feature management journey
Once you are done with the above steps, you can proceed to your hansel dashboard and start creating your first journey under the Feature Management option from the left menu.
Updated 5 months ago